Our Android Training Course equips you with the skills needed to develop robust mobile applications. You’ll learn the fundamentals of Android development, including Java/Kotlin programming, user interface design, and working with APIs. The course covers Android Studio, the key development environment, and explores advanced topics like database integration with SQLite, real-time data with Firebase, and app deployment on Google Play. Hands-on projects ensure practical experience, enabling you to build, test, and publish your own apps. By the end of the course, you’ll be proficient in creating feature-rich Android applications, ready to advance your career as a mobile developer. Ideal for beginners and those looking to refine their development skills.
Android Development
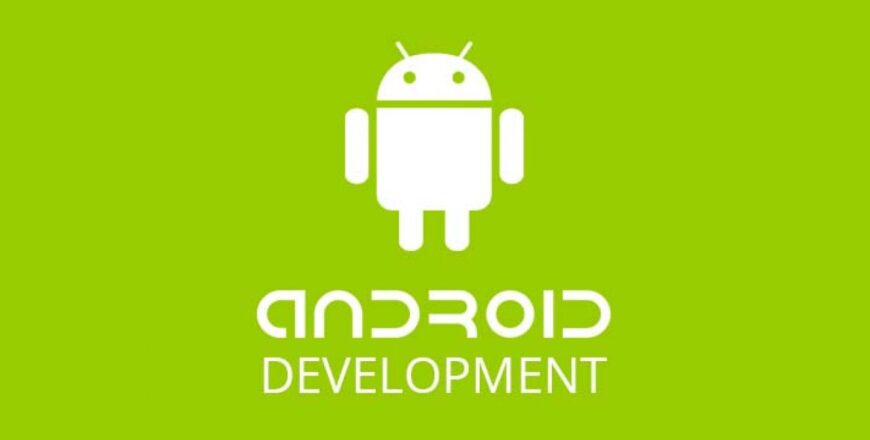
JAVA PROGRAMMING TRAINING
KEY FEATURES
- Comprehensive Curriculum: Covers basics to advanced topics in Android development.
- Programming Languages: Instruction in Java and Kotlin.
- Android Studio: Mastering the primary development environment.
- User Interface Design: Creating intuitive and responsive UIs.
- APIs and Libraries: Integrating third-party APIs and libraries.
- Database Integration: Working with SQLite and Firebase for data management.
- Real-time Data: Implementing real-time features with Firebase.
- App Deployment: Publishing apps on Google Play Store.
- Hands-on Projects: Practical experience through real-world projects.
- Best Practices: Learning coding standards and performance optimization.
- Debugging and Testing: Ensuring app reliability and performance.
- Career Support: Guidance on building a portfolio and job preparation.
- Fundamentals: Understand Android architecture and development tools.
- Languages: Master Java and Kotlin for app development.
- UI/UX Design: Create responsive and intuitive user interfaces.
- Data Handling: Integrate SQLite and Firebase for data management.
- API Integration: Implement third-party APIs for enhanced functionality.
- Real-time Features: Utilize Firebase Realtime Database for live data updates.
- Deployment: Publish apps on the Google Play Store.
- Testing and Debugging: Ensure app quality using Android Studio tools.
- Security: Apply best practices for app security.
- Performance: Optimize app performance and memory usage.
- Version Control: Manage code changes with Git.
- Projects: Apply skills through hands-on projects for practical experience.
- United States: $60,000 – $80,000 per year
- United Kingdom: £25,000 – £35,000 per year
- India: ₹300,000 – ₹600,000 per year
- Canada: CAD 50,000 – CAD 70,000 per year
- Australia: AUD 55,000 – AUD 75,000 per year
- Market Dominance: Android holds a significant global market share in the mobile operating system industry.
- Global Reach: Access to a vast and diverse user base worldwide.
- Versatility: Supports a wide range of devices from smartphones to wearables and smart TVs.
- Development Flexibility: Choice of programming languages (Java, Kotlin) and robust development tools (Android Studio).
- Integration Opportunities: Seamlessly integrates with other Google services and third-party APIs.
- Innovation Potential: Constant updates and advancements in Android OS and development frameworks.
- Career Opportunities: High demand for skilled Android developers across industries.
- Monetization: Potential for app monetization through Google Play Store and in-app purchases.
- Community Support: Active developer community for learning and collaboration.
- Continuous Growth: Opportunities for learning and evolving with new technologies and trends in mobile development.
- Design and Development: Create mobile applications for Android platforms using Java or Kotlin.
- UI/UX Design: Design intuitive and visually appealing user interfaces.
- Integration: Integrate APIs, third-party libraries, and services into applications.
- Testing and Debugging: Ensure app functionality, performance, and reliability through testing and debugging.
- Optimization: Optimize application performance and memory usage.
- Deployment: Publish applications on the Google Play Store and manage updates.
- Documentation: Maintain documentation related to app development and updates.
- Collaboration: Coordinate with cross-functional teams including designers, product managers, and QA testers.
- Continuous Learning: Stay updated with Android development trends, tools, and technologies.
- Problem Solving: Identify and resolve technical issues and challenges during development.
COURSE CONTENT
Introduction about Java
1: Introduction about Java Programming.
2: Key Features Of Java Programming Language
3: Basics of Object-Oriented Programming
4: Compiling and Running First Java Program
5: Java Data Types
6: Java Operators
7: Primitive Type Casting
8: Taking Input from Console (Using Console class)
9: constant (final variable)
Declaring Class
1: Declaring class
2: Instantiating class
3: Java memory management
4: Object and Reference
String Processing and Data Formatting
1: The immutability of Java. lang. String class
2: Key methods of the java. lang.String class
3: The java. lang.StringBuffer and java. lang.StringBuilder class
4: Dates, Numbers, Currencies, and Locale
Implementation of Inheritance & Polymorphism
1: Basics of Inheritance
2: IS-A and HAS-A Relationship
Example
3: Member access and inheritance
4: Constructor in inheritance
5: Use of super keyword
6: Calling super class constructor (super() constructor call)
7: Method Overriding (Dynamic Polymorphism)
8: final method
9: Dynamic Method Dispatch
10: Reference Variable Casting
11: Abstract class and abstract methods
Collection Framework in Java.
1: Overriding toString(),equals() and hashCode() methods from the Object class
2: Collection Framework overview
3: Important Classes And Interfaces In Collection Framework
4: Working with List
Control Statement
1: if-else statement
2: switch-case statement
3: for loop
4: while loop
5: do-while
Constructors
1: What are constructors?
2: Need for constructors
3: Declaring constructor
4: Default Constructor
Package
1: Concept of package
2: Creating user defined packages
Introduction about Array
1: Declaring, Instantiating, and Initializing 1D array
2: Concept of Array of Arrays [2D array]
3: Passing arrays to methods
4: var-args (Variable arguments)
Concept about Exception Handling.
1: Concept of Exception in Java
2: Exception class hierarchy
3: Handling exceptions using try, catch, finally
4: Use of throws clause (Propagating Exception)
5: Use of throw Clause
6: Creating User Defined Exception Class
Concept of Threading
1: Overview Of Threads
2: Defining, Instantiating And Running Thread
3: Thread Life Cycle (Thread State Transition)
4: sleep() and join() method
Android Development
Introduction about Android.
1: Introduction about Android.
2: Environment Setup Android.
3: Launch AVD in Android.
4: Introduction about Android SDK
5: Architecture of Android.
6: Introduction about API in Android?
7: Work with Android Emulator.
8: Types of Emulator Use in Android.
9: Android Versions Use in Android OS.
10: Compiling and Running Application.
11: Features of Android.
12: Android Weights.
13: About User Interface layout in Android and Introduction about XML?
Introduction About Activity and Services
1: Introduction about Activity and explain about Activity Life Cycle.
2: Introduction about Services and explain about Service Life Cycle.
3: Introduction about Broadcasting.
4: Intent in Android and Types of Intent in Android.
5: Handler in Background Threads
Introduction about Rest API (Web Services)
1: Java Script Object Notation Parsing (Json Parsing in Android).
2: Introduction About Web Services and Types of Web Services.
3: Run Time Permission Android.
4: What is .JSON and .XML parsing?
5: AsynkTask Class with Different Method.
6: Multiple Types Java Script Object Notation API.
Android Library and 3rd Party Lib
1: Fetching data from the Server using Volley Lib.
2: Get Data from the Server using Volley Lib.
3: Post Data from the Server using Volley Lib.
4: Martial Design Lib,
5: Card view.
6: Get Image from the Server using Picasso Lib
Android Weights & UI controls
1: Text View
2: Edit Text View
3: Button
4: Radio Button
5: Check Box
6: Toggle Button
7: Image Button
8: Image View
9: Progress Bar
10: Spinner
11: List View
12: Auto complete Text View
13: Grid View
14: Custom Grid view
15: Progress Bar
16: Seek Bar
17: Analog and Digital Time
Fundamental of Material Design with Animation
1: Introduction about Material Design and Types of Library in android.
2: Invention of Material Design.
3: Create Navigation Drawer Slide Menu.
4: Add Animation in Android Activity and UI controls.
Implementation of Google API on Android Mobile
1: Generate Sha1 key for Google Play Store in Android.
2: Access Google Map API key.
3: Display Google Map Android Phone
Content Provider (SQLITE DATA BASE)
1: Working with Content Provider.
2: Store Data from Mobile Device.
3: Share preferences
Deploy App on Google Play Store
1: Upload Application on Google Play Store.
2: Complete Deployment in Google Play store